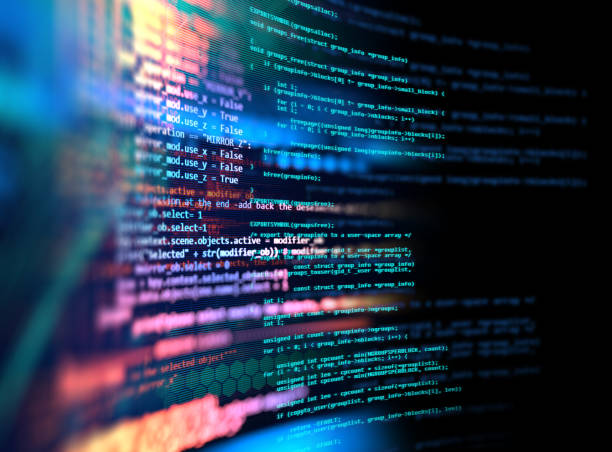
Unleashing Java’s String Permutations: Possibilities Abound
Introduction
Java, a versatile and powerful programming language, is well-known for its ability to tackle a wide range of tasks. One of its fascinating capabilities lies in string manipulation. In this comprehensive exploration of Java’s capabilities, we will delve deep into the concepts of string permutations and subsequences, understanding their significance and exploring the myriad possibilities they offer.
Understanding Subsequences of a String
A subsequence of a string is a sequence of characters that appears in the same order as they do in the original string, but not necessarily consecutively. This concept is fundamental when dealing with string manipulation and pattern recognition. Let’s delve deeper into the concept:
1. Subsequence of a String: A subsequence can be as short as one character or as long as the entire string. It is formed by selecting characters from the original string while preserving their relative order. For example, in the string “Java,” the subsequences include “J,” “a,” “v,” “a,” “Ja,” “Jv,” and “ava.”
2. Importance of Subsequences: Subsequences are crucial in various domains, from natural language processing to DNA sequence analysis. They play a vital role in tasks such as pattern matching, data compression, and even in educational games like Scrabble.
Permutation of String and Their Significance
String permutations are a fascinating concept in combinatorial mathematics and computer science. A permutation of string is any rearrangement of its characters. In Java, generating string permutations can lead to a wide array of applications, including anagrams, word games, and algorithm design. Let’s explore this concept further:
1. Permutation of String: A Permutation of String involves rearranging its characters to form a new string. For instance, the permutations of “abc” include “abc,” “acb,” “bac,” “bca,” “cab,” and “cba.”
2. Anagrams: Generating Permutation of String is vital for anagram generation. Anagrams are words or phrases formed by rearranging the letters of another word or phrase. For example, “listen” and “silent” are anagrams.
3. Algorithm Design: Permutations play a crucial role in algorithm design. Problems like the traveling salesman problem and various optimization tasks involve exploring all possible permutations of a set of elements to find the best solution.
String Permutations in Java
Now that we understand the significance of string permutations, let’s see how Java unleashes its power to generate permutations of a string.
1. Using Recursion: Recursion is a common technique to generate string permutations in Java. By recursively swapping characters at different positions, you can explore all possible permutations. Here’s a simple example of a recursive permutation generator:
“`java
public static void permute(String str, int left, int right) {
if (left == right) {
System.out.println(str);
} else {
for (int i = left; i <= right; i++) {
str = swap(str, left, i);
permute(str, left + 1, right);
str = swap(str, left, i); // Backtrack
}
}
}
public static String swap(String str, int i, int j) {
char[] charArray = str.toCharArray();
char temp = charArray[i];
charArray[i] = charArray[j];
charArray[j] = temp;
return String.valueOf(charArray);
}
“`
2. Using Libraries: Java provides libraries to handle permutations efficiently. The `Collections` class in the `java.util` package offers a convenient method to generate permutations of a collection:
“`java
import java.util.Collections;
import java.util.ArrayList;
import java.util.List;
public static List<String> generatePermutations(String input) {
List<String> permutations = new ArrayList<>();
for (int i = 0; i < input.length(); i++) {
char character = input.charAt(i);
String remaining = input.substring(0, i) + input.substring(i + 1);
List<String> subPermutations = generatePermutations(remaining);
for (String subPermutation : subPermutations) {
permutations.add(character + subPermutation);
}
}
return permutations;
}
“`
Exploring the Possibilities
String permutations open up a world of possibilities. Let’s examine a few scenarios where Java’s ability to generate permutations can be harnessed:
1. Word Games: In word games like Scrabble or crossword puzzles, generating permutations of a given set of letters can help players discover all possible words they can create.
2. Genetic Algorithms: Genetic algorithms mimic the process of natural selection and evolution to solve complex problems. Permutations play a pivotal role in generating diverse solutions and exploring the search space.
3. Algorithmic Challenges: Programming contests and algorithmic challenges often involve problems where generating permutations is a key component of the solution. Efficient permutation generation can significantly improve the performance of algorithms.
4. Anagram Detection: Detecting anagrams is a common problem in text processing. By generating permutations of a word and checking against a dictionary, you can find valid anagrams.
5. Cryptography: In cryptography, generating permutations is sometimes used to create encryption keys or perform cryptographic operations. It adds an element of randomness to cryptographic processes.
Challenges and Efficiency
While string permutations are incredibly versatile, they can be computationally intensive for large strings. Generating all permutations of a string of length `n` results in `n!` possible permutations, which grows rapidly as `n` increases. This can lead to performance challenges, especially when dealing with long strings.
Efficiency becomes a significant concern, and Java offers various approaches to mitigate these challenges:
1. Limit the Permutations: Depending on the application, you may not need all possible permutations. You can set constraints or filters to limit the number of permutations generated.
2. Use Iteration: Iterative algorithms often outperform recursive ones for generating permutations, especially for larger input strings.
3. Parallelization: In modern Java, you can harness the power of multithreading and parallelization to generate permutations more efficiently, as each permutation can be computed independently.
4. Memoization: If you’re generating permutations with repeated characters, memoization can help avoid redundant computations and improve efficiency.
Future Possibilities
The field of string permutations in Java is not static; it continues to evolve, with new possibilities emerging. Here are some future trends and possibilities to watch for:
1. GPU Acceleration: As graphics processing units (GPUs) become more accessible for general-purpose computing, utilizing GPUs for permutation generation can significantly speed up the process.
2. Machine Learning: Machine learning models can learn and predict patterns in permutations, enabling faster and more efficient generation, particularly for specific types of strings.
3. Optimized Libraries: Java libraries for permutation generation may continue to evolve, providing more efficient and user-friendly methods.
4. Quantum Computing: In the realm of quantum computing, unique algorithms and approaches for string permutations may emerge, capable of handling exponentially large problem spaces efficiently.
Conclusion
Java’s prowess in handling string permutations is a testament to its versatility as a programming language. The concepts of subsequences and permutations, while seemingly simple, offer a plethora of applications, from word games to genetic algorithms. Java’s ability to manipulate strings and generate permutations opens the door to countless possibilities.
Whether you’re a developer tackling algorithmic challenges or a gamer looking to conquer word games, understanding the power of string permutations in Java can be a game-changer. As technology continues to advance, we can only imagine the exciting developments and new frontiers that lie ahead for string manipulation and permutation generation in Java. The possibilities truly abound, and the journey has just begun.